What’s code style, and why does it matter? How do you align the code style in your project fast and cheaply? How to never discuss style during code reviews again?
I’m a Front-end Engineer at Bonial, working on migrating our main user-facing platform to the new (next.js) stack. Big fun of functional programming and TypeScript.
The typical software developer spends 70% to 90% of working time reading code, way more than writing/editing. As it’s so time-consuming, making reading more straightforward is a big deal.
One way to achieve it is to have a consistent code style among the whole codebase on a project and/or company level.
In practice, without an agreement and enforcement of code style, every software developer codes in their own manner. It can be “small” things, like the maximal length of lines, an empty line at the end of the file, etc. After a short time, the code style becomes messy – to the point that just reading a block of code might indicate the author.
Inconsistent code style can cause a headache during code reviews if the code style of two coders comes to “conflict.” So, any large codebase with multiple team members should look as if only one programmer wrote it.
Once we’re all on the same page, it’s time to implement the code style. Mostly I code in JavsScript/TypeScript, and the best option in the area is Prettier – the opinionated code formatter. Opinioned means it comes with batteries included and doesn’t give you much freedom to implement any code style you could imagine. Don’t worry – you’re in good company, as Prettier is used in companies such as Dropbox, Heroku, Spotify, and many others.
Before you even begin installing Prettier to your codebase, ask your co-workers to discuss their preferences regarding the following things:
- Maximal length of a line (of code)
- Single or double quotes for strings
- Semi columns after each expression
- Trailing commas
- Tabs or spaces for indentation
Once you have this feedback, it’s time to create in the root of your project file .prettierrc and fill it with your team’s agreements; this could look like:
{
“trailingComma”: “es5”,
“tabWidth”: 4,
“semi”: false,
“printWidth”: 100,
“singleQuote”: false
}
The whole list of options, along with their descriptions, can be found on this page.
Prettier can work as standalone, but also in conjunction with ESLint. In the article, we assume the standalone one. Let’s install it – all commands should be run in Terminal at the root of your npm project.
“`
npm install –save-dev –save-exact prettier
“`
Once it’s there, time to check your code style:
“`
npx prettier –check “components/**/*.{tsx, js}”
“`
Feel free to adjust the command above to point prettier to directories you have in your project.
Do you see any errors in your output? Don’t worry; we’ll fix them in a minute.
Time for magic!
Type the same command but replace –check with –write.
“`
npx prettier –write “components/**/*.{tsx, js}”
“`
Check out the output of the git diff command!
I hope JavaScript (and hopefully TypeScript) were formatted according to the config you’ve set up before. You could be shocked because your code became beautiful or terrible – that depends on your taste.
Let’s go to the next step. You can derive even more value from Prettier by integrating it with your code editor. I write code inside IntelliJ IDEA using the fantastic Prettier plugin. It works like magic – formatting your code just after you’ve typed it.
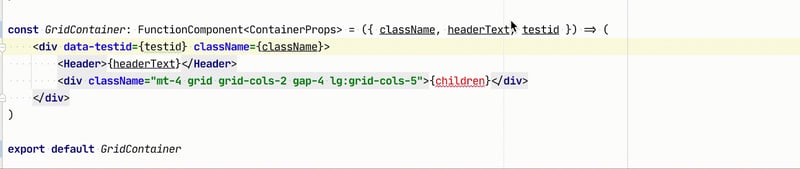
- That’s it, share the article with your co-workers, let’s bring consistent code style in our projects!
Summary
Checking code style using a Computer helps streamline the developing process, reduces the time for code reviewing, and the cognitive load to read codebases. Prettier is a terrific tool in the front-end world and formats your code as soon as you’ve typed a line. Thanks to its abilities, every team member pushes code in the same style regardless of their level and experience.
Author
This article was written by Vladislav Lisovskii, a Frontend Engineer at Bonial.